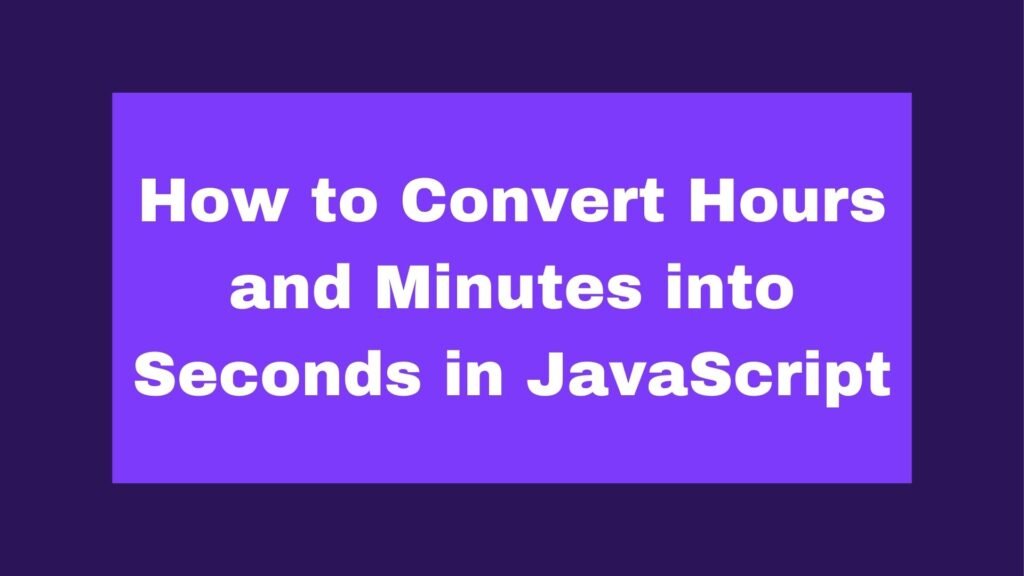
Converting hours and minutes into seconds is a common task in JavaScript, often required in scenarios like countdown timers, time calculations, and more. JavaScript provides simple arithmetic operations to achieve this conversion.
function convertToSeconds(hours, minutes) {
return (hours * 3600) + (minutes * 60);
}
let totalSeconds = convertToSeconds(2, 30); // 2 hours and 30 minutes
console.log(totalSeconds); // 9000
To convert hours and minutes into seconds in JavaScript, multiply the number of hours by 3600 (the number of seconds in an hour) and the number of minutes by 60 (the number of seconds in a minute), then sum the results:
Methods on How to Convert Hours and Minutes into Seconds in JavaScript
Time conversions are essential in many programming tasks, such as scheduling, timers, or handling user input related to time. Converting hours and minutes into seconds in JavaScript involves simple multiplication and addition, but wrapping this logic in a function can improve code clarity and reusability.
Basic Arithmetic Approach
To convert hours and minutes into seconds, you need to understand the basic relationships:
- 1 hour = 3600 seconds
- 1 minute = 60 seconds
Example 1: Basic Calculation
let hours = 2;
let minutes = 30;
let totalSeconds = (hours * 3600) + (minutes * 60);
console.log(totalSeconds); // 9000
Explanation:
hours * 3600
: Converts hours to seconds.minutes * 60
: Converts minutes to seconds.- Sum the two values: This gives the total number of seconds.
Using a Function for Reusability
Encapsulating the conversion logic in a function allows you to reuse it easily across your application.
Example 2: Conversion Function
function convertToSeconds(hours, minutes) {
return (hours * 3600) + (minutes * 60);
}
let totalSeconds = convertToSeconds(1, 45); // 1 hour and 45 minutes
console.log(totalSeconds); // 6300
Explanation:
convertToSeconds(hours, minutes)
: A function that takes two arguments (hours and minutes) and returns the total number of seconds.return (hours * 3600) + (minutes * 60);
: The function performs the conversion using basic arithmetic and returns the result.
Example Scenarios
Example 3: Converting Different Times
let time1 = convertToSeconds(0, 15); // 15 minutes
let time2 = convertToSeconds(3, 20); // 3 hours and 20 minutes
console.log(time1); // 900
console.log(time2); // 12000
Explanation:
- Different inputs: The function can handle various inputs, from zero hours to multiple hours, making it versatile.
Handling Edge Cases
Example 4: Handling Zero Values
let time3 = convertToSeconds(0, 0); // 0 hours and 0 minutes
console.log(time3); // 0
let time4 = convertToSeconds(1, 0); // 1 hour and 0 minutes
console.log(time4); // 3600
Explanation:
- Zero values: The function correctly handles cases where either hours or minutes (or both) are zero, returning the appropriate number of seconds.
Conclusion
Converting hours and minutes into seconds in JavaScript is straightforward with basic arithmetic operations. By creating a reusable function, you can simplify your code and make it more readable and maintainable. Whether you’re working with simple time calculations or more complex scenarios, understanding how to perform these conversions is a fundamental skill in JavaScript development.